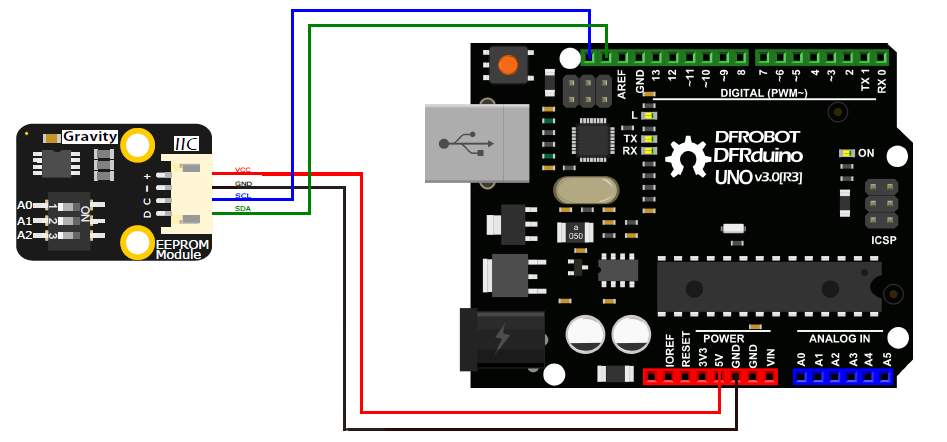
Premessa: Arduino ha una piccola quantità di spazio di archiviazione dati, Arduino UNO ha 32k.Non ha spazio sufficiente se si desidera archiviare molti dati.Pertanto, è necessario aggiungere un modulo di archiviazione dati EEPROM per aumentare lo spazio di archiviazione. Il modulo di memorizzazione dati EEPROM trasmette i dati ad Arduino UNO utilizzando il protocollo I2C. Utilizzava una EEPROM seriale CMOS I2C 24LC256 256K fornita da Microchip Techonogy Inc. 24LC256 è una memoria di sola lettura programmabile cancellabile elettricamente seriale da 32K x 8 (256Kbit). utilizzato con la scheda di schermatura dell’interfaccia di I2C on Board.
CARATTERISTICHE TECNICHE:
- Alimentazione: 2,5 V ~ 5,5 V
- Tecnologia CMOS a basso consumo
- Corrente di lettura: 400 uA max a 5,5 V, 400 kHz
- Corrente di standby: 1 uA max a 3,6 V, I-temp
- Interfaccia: protocollo I2C, interfaccia seriale a 2 fili
- EEPROM: 32K x 8 (256 Kbit)
- Dimensioni: 30,00*21,05 (mm)
- Diametro del foro di montaggio: 3,5 mm
- Temperatura ambiente con alimentazione applicata: -40℃~+125℃
- Peso: 10 g
LISTA MATERIALI:
PANORAMICA DELLA SCHEDA:
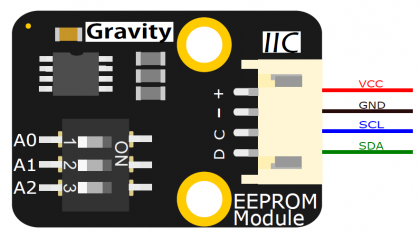
INDIRIZZO TRUE TABLE:
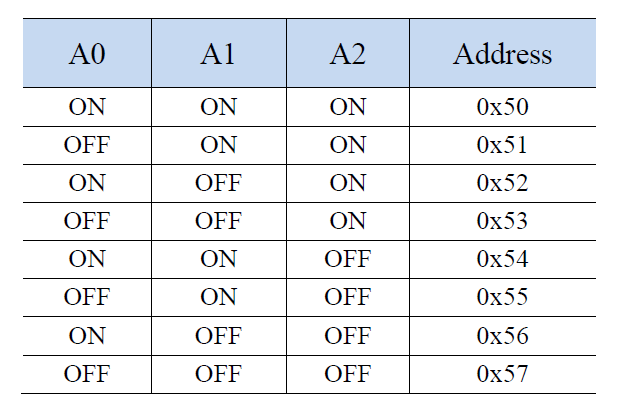
SCHEMA DI COLLEGAMENTO:
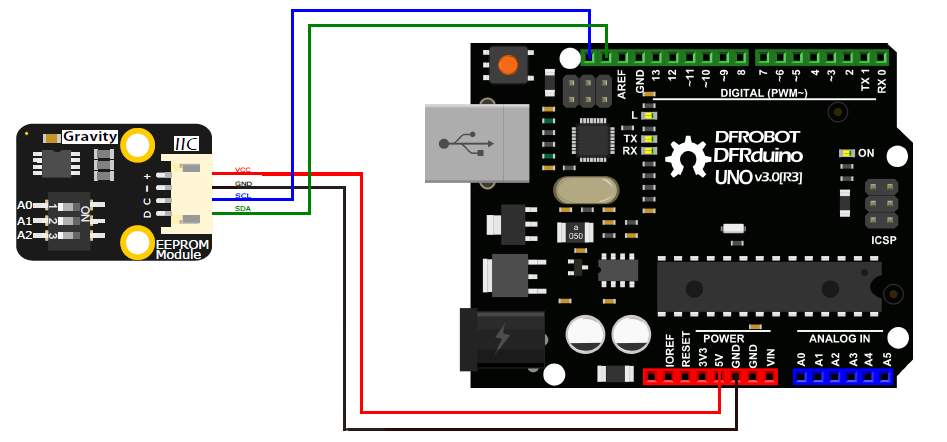
CODICE DI ESEMPIO:
Librerie:
- Wire LGPL: arduino.cc/en/Reference/Wire
#include <Wire.h> //I2C library
void i2c_eeprom_write_byte( int deviceaddress, unsigned int eeaddress, byte data ) {
int rdata = data;
Wire.beginTransmission(deviceaddress);
Wire.write((int)(eeaddress >> 8)); // MSB
Wire.write((int)(eeaddress & 0xFF)); // LSB
Wire.write(rdata);
Wire.endTransmission();
}
// WARNING: address is a page address, 6-bit end will wrap around
// also, data can be maximum of about 30 bytes, because the Wire library has a buffer of 32 bytes
void i2c_eeprom_write_page( int deviceaddress, unsigned int eeaddresspage, byte* data, byte length ) {
Wire.beginTransmission(deviceaddress);
Wire.write((int)(eeaddresspage >> 8)); // MSB
Wire.write((int)(eeaddresspage & 0xFF)); // LSB
byte c;
for ( c = 0; c < length; c++)
Wire.write(data[c]);
Wire.endTransmission();
}
byte i2c_eeprom_read_byte( int deviceaddress, unsigned int eeaddress ) {
byte rdata = 0xFF;
Wire.beginTransmission(deviceaddress);
Wire.write((int)(eeaddress >> 8)); // MSB
Wire.write((int)(eeaddress & 0xFF)); // LSB
Wire.endTransmission();
Wire.requestFrom(deviceaddress,1);
if (Wire.available()) rdata = Wire.read();
return rdata;
}
// maybe let's not read more than 30 or 32 bytes at a time!
void i2c_eeprom_read_buffer( int deviceaddress, unsigned int eeaddress, byte *buffer, int length ) {
Wire.beginTransmission(deviceaddress);
Wire.write((int)(eeaddress >> 8)); // MSB
Wire.write((int)(eeaddress & 0xFF)); // LSB
Wire.endTransmission();
Wire.requestFrom(deviceaddress,length);
int c = 0;
for ( c = 0; c < length; c++ )
if (Wire.available()) buffer[c] = Wire.read();
}
void setup()
{
char somedata[] = "this is data from the eeprom"; // data to write
Wire.begin(); // initialise the connection
Serial.begin(9600);
i2c_eeprom_write_page(0x50, 0, (byte *)somedata, sizeof(somedata)); // write to EEPROM
delay(10); //add a small delay
Serial.println("Memory written");
}
void loop()
{
int addr=0; //first address
byte b = i2c_eeprom_read_byte(0x50, 0); // access the first address from the memory
while (b!=0)
{
Serial.print((char)b); //print content to serial port
addr++; //increase address
b = i2c_eeprom_read_byte(0x50, addr); //access an address from the memory
}
Serial.println(" ");
delay(2000);
}
Buon progetto.